What is a Geolocation API?
An API is a programming interface for an application; in other words, it is the set of functions that a programmer can use to expose structured data provided by the publisher of the API.
A geolocation API is one that provides a way for programmers to use a computer's Internet address, for example, to obtain geographic info such as the user's location.
The result of a geolocation API might provide:
- bearing and range from a known landmark,
- latitude and longitude coordinates,
- altitude, speed, and heading,
- accuracy of the data.
Client-side Geolocation
In the client / server paradigm a client is an application that sends requests to and receives responses from a server. One of the most common client applications and the one we will be referring to in this article is the trusty web browser. Supported examples include: Chrome, Firefox, Opera, Safari and Internet explorer.
As opposed to a server-side geolocation API which requires the client to send requests to an external server, a client-side geolocation API uses an internal script to access location information.
Geolocation API
The W3C Geolocation API defines a set of ECMAScript standard objects to give the client's device location through the consulting of Location Information Servers. The specification document was first published to GitHub in 2008.
Note: On most devices the geolocation API feature is secure-only meaning that it will only work with an encrypted transport layer, for example HTTPS (not HTTP).
This is all done on the client-side; therefore, no server round-trip is made which results in fast response times.
Currently all modern browsers support the geolocation API: (source)
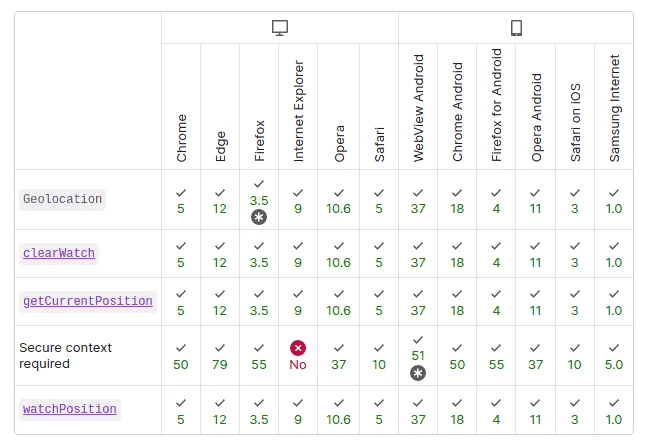
Geolocation API Examples
Let's look at some examples.
JavaScript API Example
The quickest way to test a geolocation API is to use the geolocation API services built into your web browser. For this tutorial section we will be executing some JavaScript inside of the web developer tools console.
If you don't know how to run JavaScript then just come along for the ride or skip to the next section where we use the IP geolocation API from Abstract API.
Web browsers were originally designed to be secure to prevent unwanted attacks from a rogue script that might try to execute harmful code on our machine or to access private information. A W3C standards-compliant app requires a secure connection (HTTPS) and will not make any location information available without the user's explicit permission.
Step 1: The Console
Start your browser up in a new tab and open the console so we can start entering some code.
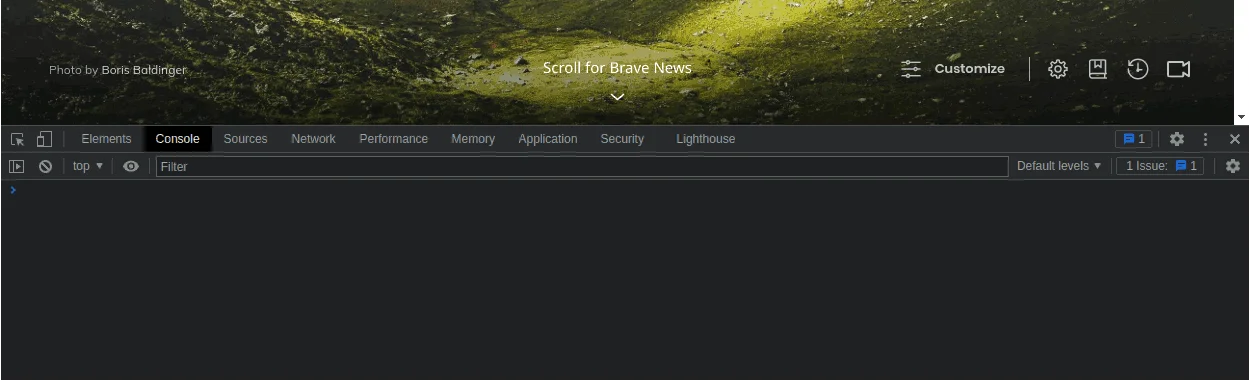
Click in the console near the alligator prompt:
Note: Your prompt may be different depending on which browser you use.
Step 2: Verify API Support
The geolocation API in the browser exposes the geolocation object of the navigator. Calling the getCurrentPosition function will provide the position data including an accuracy value for how good the data is depending on the location information sources available.
Make sure you're in a brand new tab with a blank URL and not on a website.
Let's first display our user agent to make sure we have access to the navigator object.
Enter the following into the console and press enter:
It should return a string something like: 'Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/99.0.4844.51 Safari/537.36'.
Let's make sure the geolocation object is supported. Enter the following:
If you got the value true then your browser is supported.
Step 3: Get Current Location
Let's query the browser for our location data by writing a small "one-liner" script to execute the getCurrentPosition function which accepts a separate "callback" function as the argument between the parentheses.
To obtain the user's current location, you can call the getCurrentPosition() method. This initiates an asynchronous request to detect the user's position, and queries the positioning hardware to get up-to-date information. When the position is determined, the defined callback function is executed. --MDN
The getCurrentPosition function will call our callback function with the location data it finds. In our example it will pass the position information to console.log which will print it to the console.
Enter the following code into the console:
The console should display a GeolocationPosition object:
By default getCurrentPosition will try to answer as fast as possible using readily available information such as network address. It is possible to pass getCurrentPosition an optional third parameter with the enableHighAccuracy member if you want to obtain higher accuracy at the cost of slower response times or increased power consumption.
The enableHighAccuracy member provides a hint that the application would like to receive the most accurate location data. The intended purpose of this member is to allow applications to inform the implementation that they do not require high accuracy geolocation fixes and, therefore, the implementation MAY avoid using geolocation providers that consume a significant amount of power (e.g., GPS). --W3C
Step 4: Asking User's Permission
Wait! My browser didn't ask for my permission to reveal my current position!
If you opened your browser to a new tab to execute your JavaScript code then it assumes you are the author and no permission verification is made.
Now go to a website, for example abstractapi.com, and execute the same code in the console and see what happens. The browser should popup a dialog that informs you that the website wants to know your device's location.
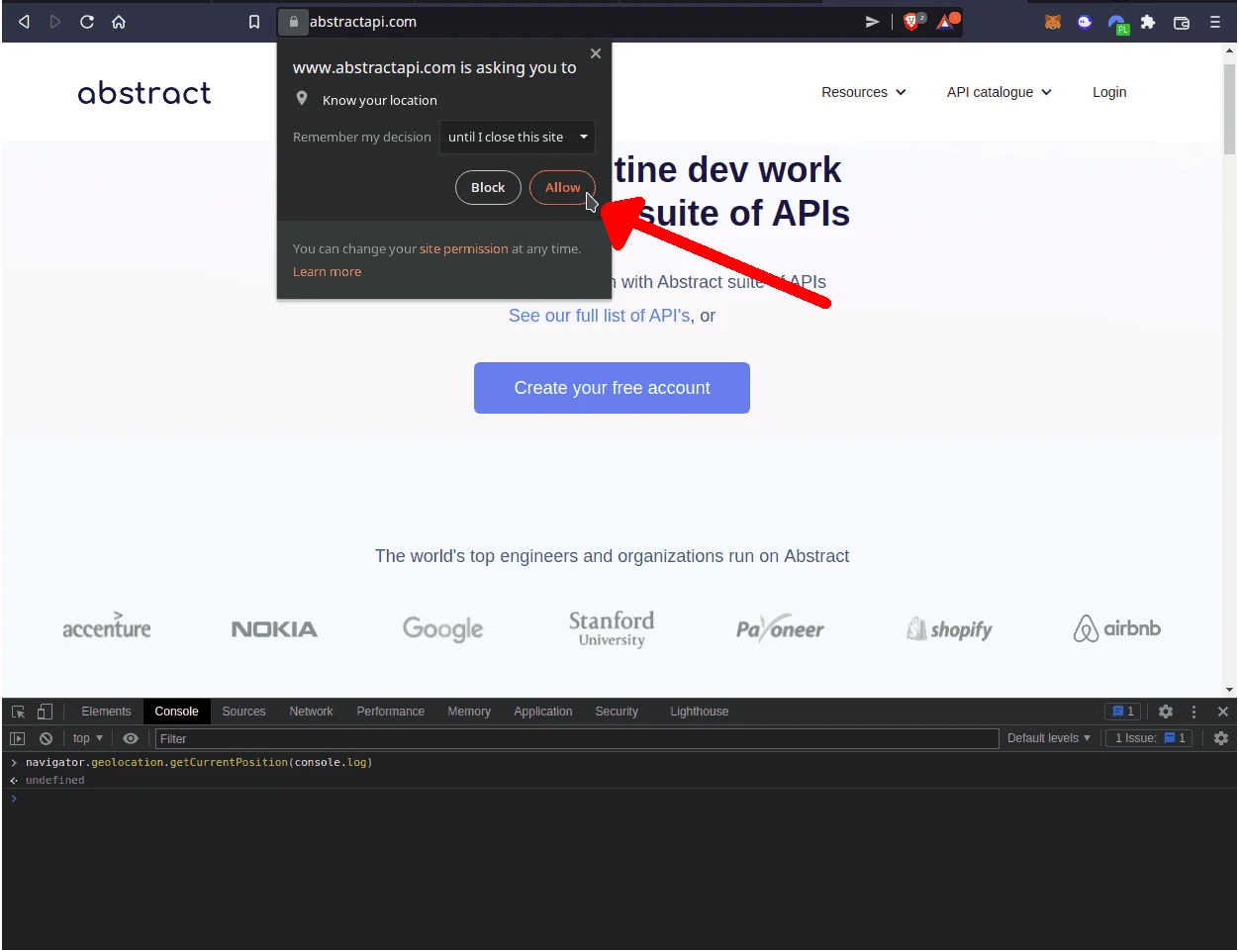
IP Geolocation API at Abstract
The simplest way to test a geolocation API is to use an online API service.
For this tutorial section, we will be using the function set provided by Abstract API.
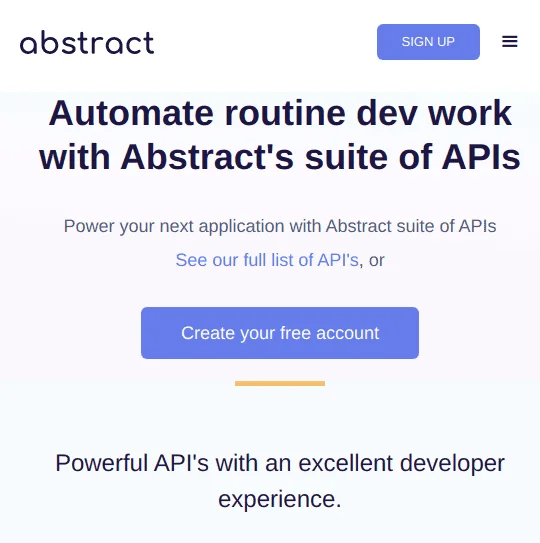
Step 1: Sign-up
Sign up for a free account at https://app.abstractapi.com/users/signup.

Step 2: Call the API
Head to the IP geolocation API tester.

Press the blue Make test request button and you should get back your current position information in JSON format:
Latitude and longitude
Notice among the results we have the latitude and longitude coordinates which an app could use to plot the user's location on a map. This is how Google Maps and others plot your current location by using a similar geolocation API.
Step 3. Save API Key
Jot down your API Key as we will need it in the next example.

Your key should look something like the following:
Python API Example
In the previous examples we first worked with script on the client-side. Then we went to the Abstract API website to make a request to the server-side. Now we will use both: the client-side and the server-side.
Emma Jagger's article How to geolocate an IP address in Python contains more information.
In this tutorial we will be using the Python programming language. And the version we will be using is Python 3.
You can see if you have Python 3 installed by executing the python binary in a shell:
It should display something like "Python 3.10.2" which contains a three (3) as the first number which means that I am running Python version 3.
Note: If you don't have Python 3 installed you can search for an online REPL.
Part 1: Interpreter
Python code can run as a script from within a file.
Or in an interactive session:
Note: Prompts legend:
- $ - The dollar sign is used to denote a user command-line prompt.
- >>> - The triple alligator used to denote the interactive Python prompt.
Most systems expose a Python interpreter by typing python from the command line.
Part 2: Ipinfo.io
While you can certainly execute the following code in a script file; in this part, we are prefixing each line with the interactive prompt (>>>) to distinguish them from the non-prefixed lines of output.
Open an interactive Python interpreter session.
Let's make a generic unauthorized request to ipinfo.io.
Try this "two-liner" script from the Python standard library:
It should show your current location data including the loc field which represents your location latitude longitude coordinates:
If your interpreter doesn't work with this code and you have the requests library installed you can try this script:
It should give you the same results.
Of course your geolocation data will probably be different.
Part 3: Abstract API
While you can certainly execute the following code in an interactive session; in this part, we are not prefixing each line with the interactive prompt (>>>) because it might best run in a script file.
We'll use Abstract API again but let's break up the code into smaller, more manageable chunks.
Step 1: Editor
Open your favorite text editor and start entering statements as below.
Step 2: Import Function
First import the urlopen function:
Step 3: Store API Key
Next store our Abstract API key that we got from our last example:
Use your own key because the one below will not work.
Step 4: Build URL
Let's build our URL as a formatted string with the key we just stored:
Notice the f before the string which allows us to reference key within it.
Step 5: Prepare Response
Create a response object to control our data flow:
Step 6: Make Request
Now let's make the request and store the response:
Step 7: Inspect Response
Let's inspect the response:
print(type(response_bytes))This should print the class of our variable: <class 'bytes'>.
Step 8: Convert to JSON
Confirm the response content type that the server said it was going to send us:
It should print 'application/json'.
Now let's convert our response from bytes to text by decoding it:
Step 9: Inspect Text
Print the decoded response text:
The output should be a JSON formatted text string like: '{"ip_address":"75.39.85.160"...'.
Step 10: Run Script
Putting it all together:
Save file as abstract.py and execute it on the command line:
This should give us something like the following but with the position data from your actual location:
Wrapping Up
We looked at Geolocation API basics for determining location from the client-side in the browser and to the server-side from the command line. In our examples we used JavaScript and Python script to make calls. We saw the raw data coming back from our requests and now maybe we know a little more about how Google maps works.
Emma Jagger's article How to geolocate an IP address in Python contains more information.
FAQ
How do I use Geolocation API?
From the client side: "The Geolocation API is accessed via a call to navigator. geolocation ; this will cause the user's browser to ask them for permission to access their location data. If they accept, then the browser will use the best available functionality on the device to access this information..." --MDN › Web APIs
What does Geolocation API do?
The Geolocation API returns a location and accuracy radius based on information about cell towers and WiFi nodes that the mobile client can detect. --Google Developers
Is Geolocation API free?
There are many ways to get free geolocation data; for example: AbstractAPI, RapidAPI, IPWhois, IPInfo and even your own browser.