Basics of IP Geolocation
What is an IP Address?
The IP Address is a form of identification mechanism for the devices communicating over the Internet. IP addresses are defined as part of the IETF (Internet Engineering Task Force) specifications. All devices connected to the Internet are assigned a unique public IP address.
How does IP Geolocation Work?
The IP address allocation is governed by Regional Internet Registries (RIR) and Internet Assigned Numbers Authority (IANA). The RIRs are divided into five registries covering the world's major continents and geographical regions, and different IP address series are assigned to these RIRs. Further, under each RIR, multiple Internet Service Providers (ISP) operate locally, covering countries and cities. A subset of the RIR’s IP addresses is allocated to the ISPs.
When a person is connected to the Internet, their traffic is uniquely identified by their ISP's assigned IP address. Since that IP address is mapped to an RIR and a local ISP, it is possible to predict the person's location. This registry information maintained at RIRs and ISPs is the fundamental basis of IP geolocation. This is just an approximate location, as the precise location cannot be determined based on the registry information. However, ISPs maintain their internal database containing the customers' postal addresses, which can yield more accurate IP geolocation information.
Understanding Geolocation Data
The geolocation IP data mainly contains the latitude and longitude of the location of the Internet subscriber. This information is further augmented with geo-political information identifying the country code, state, province, or city.
Apart from the location-specific information, the geolocation data may also contain the ISP details, timezone, and network or connectivity-related information.
Getting Started with PHP IP Geolocation
Since most activities in the virtual world happen via websites and web applications, it is obvious that many web servers capture the IP geolocation information of the site visitor's IP address. PHP is one of the most popular programming languages for building web server logic. Therefore, we have restricted our focus to PHP’s way of finding and leveraging IP geolocation.
For retrieving the IP geolocation of a computer via PHP, it is important to connect to a central database to retrieve IP data that contains the latest IP address registry mappings from RIRs and ISP.
How to retrieve a user's IP address in PHP
The PHP variable $_SERVER is an array whose entries are created by the webserver. It contains information about the server and the HTTP connection, such as HTTP headers and the client's IP address.
The easiest way to get the visitor's IP address is to use the REMOTE_ADDR entry, which returns the client's IP address requesting the current page.
But when the webserver is behind a proxy, REMOTE_ADDR will return the proxy's IP address, not the user's. In this case, the proxy would have set the real client's IP address in an HTTP header, generally HTTP_CLIENT_IP or HTTP_X_FORWARDED_FOR. Here is a script that covers most of the cases:
How to Geolocate an IP Address in PHP with an API
This is also possible using an API service that maintains such a database.
Let’s see a quick demo of this operation using the AbstractAPI IP Geolocation API:
1 - Sign up for AbstractAPI's free IP Geolocation API
Signup for a free AbstractAPI account. After that, navigate to the IP Geolocation API page and note the API key. You can also check the documentation to get the API endpoint.
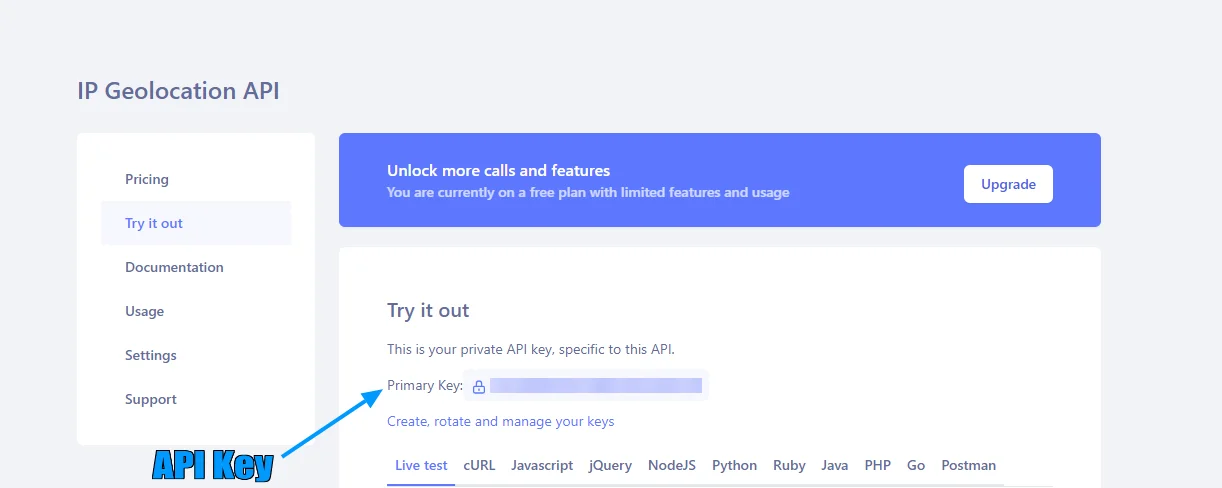
2 - Extract an IP address with the API using the following script
The Geolocation details on an IP address can be extracted by calling this API through a simple PHP script using the cURL library. Here is the complete code for the PHP script:
This code will echo country and other geo-political details associated with the location of an IP address. This code can be copied on a PHP code editor. Before saving it, replace the placeholder <YOUR_API_KEY> with the API key assigned during signup.
3 - Execute the PHP Script
To execute this PHP script, the following prerequisites are needed:
- PHP 8 runtime environment installed on the computer
- cURL PHP library is enabled through php.ini config file
The script calls the init_curl( ) PHP function to pass the IP address passed as an argument to a command line terminal to convert API JSON response in a pretty display format.

The above example displays the API response with geolocation details for the IP address 223.34.4.27. It can be seen that this IP address belongs to South Korea, and the other relevant location details like country name and region code can be analyzed further.
The logic within this PHP script can be ported to a PHP web server to detect the geolocation information of web visitors.
Another way: Using the free MaxMind IP geolocation tool in PHP
MaxMind offers multiple IP geolocation databases that can be downloaded for free from its website. The 2 main free databases are GeoLite2 Country which allows you to get the country name corresponding to an IP address, and GeoLite2 City to obtain the country, region, and city name. The second is the most accurate but also the largest in terms of file size.
The first steps are to sign-up to the MaxMind website and validate your email address. Then you will be able to download the compressed databases from your account page. You will find the geolocation database in the compressed file, named GeoLite-Country.mmdb or GeoLite2-City.mmdb.
Then you will need to download the API used to query the MaxMind database. For PHP, the officially supported API can be found on GitHub, as well as its documentation.
Installation of GeoIP2 PHP API can be done through Composer. First, install Composer if it’s not already available on your system:
Then you can install the API and its dependencies:
To finally use this API, you must create a new \GeoIp2\Database\Reader object, passing the path to the MaxMind database file downloaded earlier, then you can query it. Here is an example of its usage with the City database:
Drawbacks of using MaxMind geolocation in PHP
Downloading the MaxMind database and installing its API are additional steps that may put off some of us. Since such a database is updated regularly, you will have to download it again on your servers each time it is updated.
Moreover, the MaxMind files being quite large, you may have a performance loss when several visitors are connected simultaneously on your server.
Best IP Geolocation APIs
There are quite a few IP Geolocation APIs that strive to maintain the most accurate and detailed information about IP address locations. Here is a list of the popular APIs to choose from.
- Abstract API: Abstract IP Geolocation API provides highly accurate geolocation data covering 1.75+ million locations across 225,000+ cities worldwide. It offers a freemium subscription starting with 20,000 free API calls per month and $9/month.
- MaxMind Geo IP2 - The MaxMind GeoIP2 is a suite of libraries for building IP geolocation applications. It offers intelligent features to detect proxy and anonymous IP addresses to protect enterprise applications accessed by malicious actors. It is available under a commercial licensing model.
- IP2Location: IP2ocation offers many databases containing combinations of IP geolocation data elements classified based on country, city, and further. The data is either available as a direct download or accessible through SDKs. Each classification of IP geolocation data has separate pricing.
- GeoPlugin: It is a simple and free geolocation API that provides all the essential information related to IP geolocation. It supports easy integration with client-side JavaScript and server-side.
- HostIP: A community-based IP geolocation service with a simple API to retrieve IP geolocation data. It also offers to download its IP geolocation database. It is a totally free service.
Use Cases for IP Geolocation
Beyond the location identification and tracking of devices, IP Geolocation assumes a lot of importance in building intelligent web applications for achieving advanced capabilities such as:
- Location-specific customization in web applications: Visitors accessing the Internet from specific locations are served geotargeted content, such as local adverts, and displayed in the local language.
- Location-based filtering: Applications hosted for specific geographic locations can use geolocation data to allow or deny access to visitors based on their geolocation data.
- Cyber security Enhancement: IP Geolocation information serves to aid in cybersecurity-related scrutinies to detect fraudulent activities performed by users and tag their IP addresses and locations for further analysis.
Tips and Tricks for IP Geolocation
Accessing IP Geolocation is quite simple, but working with this data entails some responsibilities to ensure optimal performance and data usage. Here are some tips and tricks to remember while incorporating IP geolocation in a web application:
- Optimizing Geolocation Performance: It is possible to cache and build a separate local database of IP geolocation data from the APIs. This helps optimize the IP geolocation queries and saves costs in API calls.
- Handling errors in geolocation data: Geolocation data may be prone to errors depending on the API service tiers and the data quality captured by the data providers. Usually, free or community services will be prone to errors or incomplete information. To avoid issues related to IP geolocation in production-grade applications, paying subscriptions for two API services, one as primary and the other as a backup service, is advisable for fetching IP geolocation details.
- Legal use of IP Geolocation data: Applications using IP geolocation must adhere to the terms of use policies of the APIs and database providers. Further, some laws and jurisdictions consider the IP address as Personally Identifiable Information (PII). Therefore, application providers should do their due diligence if they plan to cache for store the IP addresses themselves.
- Managing risks associated with IP geolocation data capture: IP geolocation data is approximate information. It can never pinpoint the exact location of the user’s computer. Moreover, in mobile internet access, the user’s location changes constantly. Therefore, using this information only to capture the broader geo-political information is advisable.
Future of IP Geolocation:
IP geolocation data is based on broader regions and geo-political identities. This is mostly static geolocation data and only changes sometimes. However, it doesn’t address some of the more modern usage scenarios. As a result, there is a need to enhance geolocation data accuracy.
One of the ways to achieve a more accurate geolocation data capture is to rely on GPS systems. This is all the more applicable for mobile devices, as all modern smartphones have a built-in GPS mechanism. With the help of GPS, it is possible to build location-aware applications, which is otherwise impossible using the IP geolocation data available from APIs and database services. One example is a location-based reminder service, wherein a mobile app tracks the user's movement and raises an alert when the user’s phone is near a preset location.
IP geolocation also assumes more importance in the current scenario, where many AI agents and chatbots are available online. These virtual beings are constantly interacting with human beings. In such cases, capturing the IP geolocation data of computers hosting these virtual entities is important to safeguard humans in cyberspace.
Conclusion
IP geolocation continues to be an integral part of Internet operations. With technological advancements and more complex usage scenarios, geolocation-capturing techniques have evolved.
For capturing the broader geographical details of an IP address, it is good to rely on a credible API for fetching geolocation data. Geo-location capture must be done for mobile applications using GPS or A-GPS technology. However, it must be noted that capturing the geolocation through GPS is not considered a true IP geolocation technique since the GPS system does not use the IP address to find the location of the device. But for building a custom location-based application, it might be worthwhile to map the device’s IP address with GPS-captured location data to build a location-centric profile of the device for specific use cases.
FAQs
What is IP Geolocation, and why is it important?
IP geolocation is mapping an IP address to a geographical location. This mapping is done at the regional internet registry level, and further allocated at the internet service provider level, to assign IP addresses to individual internet subscribers. The IP geolocation data contains the latitude and longitude point of the IP address, the geo-political information, and ISP and connectivity details. There are many credible data sources of this information, which are offered as APIs or databases. AbstractAPI IP Geolocation API is one service that can fetch IP geolocation data from ISPs and other data providers.
How does PHP IP Geolocation work?
Every client application that sends a request to the PHP server backend also passes the client’s IP address. The PHP backend can leverage one of the Geolocation APIs or SDKs to get the geolocation data. Using the cURL library, it is possible to make an API call to an IP geolocation service to get the geolocation details for further processing. AbstractAPI is one of the most reliable services for providing IP geolocation data, from across 225,000 cities around the world.
Which PHP Geolocation library is best?
If you are looking for a simple REST API with reliable IP geolocation data, you can choose AbstractAPI IP Geolocation API, which supports IPv4 and IPv6 addresses. If you are looking for more detailed information on anonymous IP addresses or additional intelligence about the IP addresses, then you can opt for MaxMind GeoIP service. For free API access or community-supported IP address databases, you can choose between GeoPlugin or Host IP service.